4. Customizing UI
In this section, you will make some improvements to the appearance of the application:
-
Add a new column called
lastModifiedBy
to the WebDAV document wrapper data grid. This column will display the author of the latest changes made to the WebDAV file. -
Add a
lastModifiedDate
column, whose cells will show the date of the latest changes to the WebDAV file. -
Add a Download button above the data grid. Using this button, users with the Employee role will be able to download files from the knowledge base without the ability to modify them on the WebDAV server.
Using Renderers in Data Grid
In the data grid, we will learn how to display columns that are not directly linked to attributes of the associated entity. These columns are often used to show derived information. To create a column of this type, you need to define a column with a key
attribute in the XML descriptor and then generate a renderer for that column.
Adding lastModifiedBy Column
Let’s configure the display of the author of the latest changes to the WebDAV file.
Add a new column to webdavDocumentWrappersDataGrid
:
<dataGrid id="webdavDocumentWrappersDataGrid" ...>
<columns resizable="true">
<!--..-->
<column key="lastModifiedBy" header="msg://column.lastModifiedBy"/>
</columns>
</dataGrid>
This column is not bound to an entity attribute, so it has the key
attribute instead of property
.
Select the lastModifiedBy
column, switch to the Handlers tab of the component inspector and create the renderer
handler method:
@Supply(to = "webdavDocumentWrappersDataGrid.lastModifiedBy", subject = "renderer")
private Renderer<WebdavDocumentWrapper> webdavDocumentWrappersDataGridLastModifiedByRenderer() {
return null;
}
Implement the webdavDocumentWrappersDataGridLastModifiedByRenderer
method:
@Supply(to = "webdavDocumentWrappersDataGrid.lastModifiedBy", subject = "renderer")
private Renderer<WebdavDocumentWrapper> webdavDocumentWrappersDataGridLastModifiedByRenderer() {
return new TextRenderer<>(documentWrapper -> { (1)
WebdavDocument webdavDocument = documentWrapper.getWebdavDocument();
if (webdavDocument == null) {
return null;
}
WebdavDocumentVersion lastVersion = webdavDocument.getLastVersion();
return lastVersion.getCreatedBy(); (2)
});
}
1 | The method returns a TextRenderer object. |
2 | The renderer returns the name of the user who made changes to the latest version of the WebDAV document. |
Adding lastModifiedDate Column
In the lastModifiedDate
column, let’s configure the display of the date of the latest changes to the WebDAV file.
Add a new column to webdavDocumentWrappersDataGrid
:
<dataGrid id="webdavDocumentWrappersDataGrid" ...>
<columns resizable="true">
<!--..-->
<column key="lastModifiedDate" header="msg://column.lastModifiedDate"/>
</columns>
</dataGrid>
This column is not bound to an entity attribute, so it has the key
attribute instead of property
.
Select the lastModifiedDate
column, switch to the Handlers tab of the component inspector and create the renderer
handler method:
@Autowired
private ApplicationContext applicationContext;
@Supply(to = "webdavDocumentWrappersDataGrid.lastModifiedDate", subject = "renderer")
private Renderer<WebdavDocumentWrapper> webdavDocumentWrappersDataGridLastModifiedDateRenderer() {
DateFormatter dateFormatter = applicationContext.getBean(DateFormatter.class);
return new TextRenderer<>(documentWrapper -> {
WebdavDocument webdavDocument = documentWrapper.getWebdavDocument();
if (webdavDocument == null) {
return null;
}
WebdavDocumentVersion lastVersion = webdavDocument.getLastVersion();
Date lastModifiedDate = lastVersion.getCreatedDate();
dateFormatter.setFormat("MMM dd, yyyy");
return dateFormatter.apply(lastModifiedDate);
});
}
Adding Download Button
Let’s add a Download button to the WebDAV documents data grid. Using this button, users with the Employees role would be able to force a download of the selected WebDAV file.
First add a download
action to webdavDocumentWrappersDataGrid
. Then, in the buttonsPanel
hbox
, add a button and associate it with the newly created action.
<vbox expand="webdavDocumentWrappersDataGrid">
<hbox id="buttonsPanel" classNames="buttons-panel">
<!--...-->
<button id="downloadBtn" action="webdavDocumentWrappersDataGrid.download" icon="vaadin:download"/>
</hbox>
<dataGrid width="100%" id="webdavDocumentWrappersDataGrid" dataContainer="webdavDocumentWrappersDc">
<actions>
<!--...-->
<action id="download" text="msg://download" type="list_itemTracking"/> (1)
</actions>
<!--...-->
</dataGrid>
<!--...-->
</vbox>
1 | The list_itemTracking action will automatically become active only when a specific item is selected in the associated dataGrid . |
Generate an ActionPerformedEvent
handler method for the download
action. Add the logic to it:
@ViewComponent
private DataGrid<WebdavDocumentWrapper> webdavDocumentWrappersDataGrid;
@Autowired
private Downloader downloader; (1)
@Subscribe("webdavDocumentWrappersDataGrid.download")
public void onWebdavDocumentWrappersDataGridDownload(final ActionPerformedEvent event) {
WebdavDocumentWrapper webdavDocumentWrapper = webdavDocumentWrappersDataGrid.getSingleSelectedItem();
if (webdavDocumentWrapper == null) {
return;
}
WebdavDocument webdavDocument = webdavDocumentWrapper.getWebdavDocument();
if (webdavDocument == null) {
return;
}
WebdavDocumentVersion lastVersion = webdavDocument.getLastVersion(); (2)
FileRef fileReference = lastVersion.getFileReference(); (3)
downloader.download(fileReference); (4)
}
1 | Use the Downloader bean to download files. |
2 | Get the last of WebdavDocumentVersion instance - specific version of a document managed within the WebDAV system. |
3 | The returned FileRef object is a reference to the file in the file storage. |
4 | The download() method accepts the FileRef value and takes the file from the file storage specified in the FileRef object. The name and type of the file are also encoded in FileRef , so the web browser correctly chooses whether to download or display the file. |
Running the Application
Navigate to https://localhost:8443
using your web browser and sign in to the application with the credentials admin/admin
.
Choose the Knowledge base
item from the Application
menu.
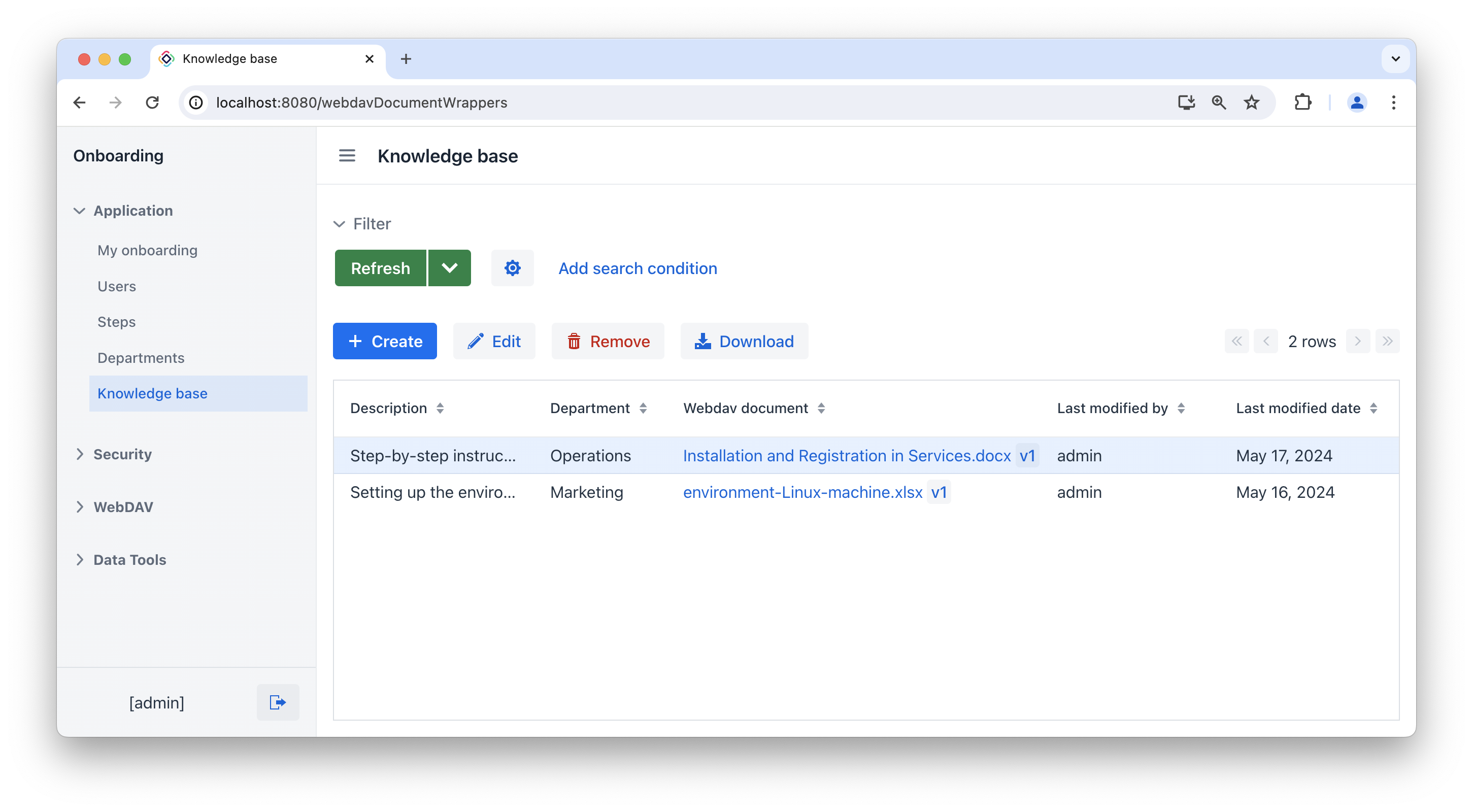
The Last modified by column displays the author of the latest modifications to the WebDav file, while the Last modified date column shows the date of the last modifications to the WebDav file.
The Download button allows users to download the selected WebDAV document.
Summary
In this section, you have learned that:
-
The data in data grid columns can be presented using renderers. Renderers provide a way to customize how the data is displayed in each column of a data grid.
-
The Downloader bean is used to download files.