Formatter
Basics
Formatter
is designed to convert some value to its string representation.
In a screen XML-descriptor, such component formatters can be defined in a nested formatter
element.
To add a nested |
Below is an example of adding a formatter to the Table.Column
component:
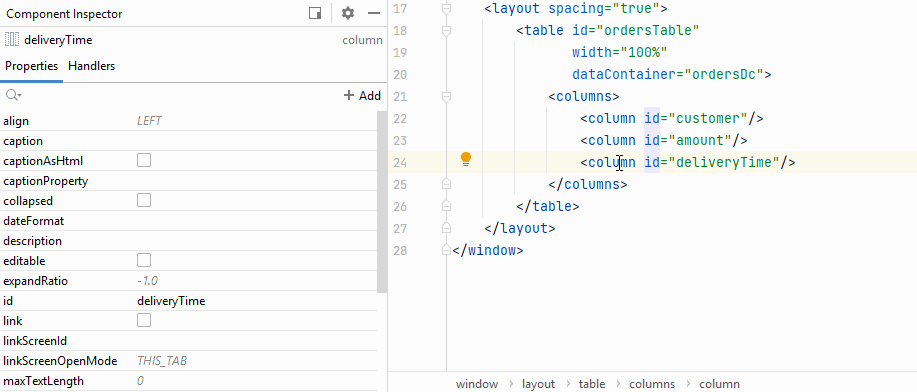
The framework contains the set of implementations for the most frequently used formatters, which can be used in your project.
Each formatter is a Prototype Bean
, and if you want to use formatters from Java code, you need to get them using ApplicationContext
.
A formatter class can be assigned to a component programmatically by submitting a formatter instance into the component’s setFormatter()
method.
Example of setting a formatter programmatically in a screen controller see in Number Formatter.
Number Formatter
Number formatter is used to format the Number
value into a string depending on the format string.
It has the following attribute:
-
format
- sets the format string describing the number format, which will be used to create theDecimalFormat
instance. It can be either a format string or a key in the message bundle.
Layout descriptor usage:
<table id="ordersTable"
width="100%"
dataContainer="ordersDc">
<columns>
<column id="amount">
<formatter>
<number format="#,##0.00"/>
</formatter>
</column>
</columns>
</table>
Java code usage:
@Autowired
protected ApplicationContext applicationContext;
@Autowired
protected Table<Order> ordersTable1;
@Subscribe
protected void onInit(InitEvent event) {
NumberFormatter formatter = applicationContext.getBean(NumberFormatter.class); (1)
formatter.setFormat("#,##0.00");
ordersTable1.getColumn("amount").setFormatter(formatter);
}
1 | Get the NumberFormatter instance using ApplicationContext . |
Date and Time Formatter
Date formatter is used to format the Date
value into a string depending on the format string.
It has the following attributes:
-
format
- sets the format string describing the date format, which will be used to create theSimpleDateFormat
instance. It can be either a format string or a key in the message bundle. -
type
- sets the formatter type, which can have aDATE
orDATETIME
value. If it is specified, the value will be formatted usingDateDatatype
orDateTimeDatatype
, respectively. -
useUserTimezone
- sets whether the formatter should display the date and time in the current user’s timezone. By default,DateFormatter
displays the date and time in the server timezone. To show the current user’s timezone, settrue
for theuseUserTimezone
attribute of the formatter.
Layout descriptor usage:
<table id="ordersTable"
width="100%"
dataContainer="ordersDc">
<columns>
<column id="deliveryTime">
<formatter>
<date format="h:mm a"/>
</formatter>
</column>
</columns>
</table>
Java code usage:
@Autowired
protected ApplicationContext applicationContext;
@Autowired
protected Table<Order> ordersTable1;
@Subscribe
protected void onInit(InitEvent event) {
DateFormatter dateFormatter = applicationContext.getBean(DateFormatter.class);
dateFormatter.setFormat("h:mm a");
ordersTable1.getColumn("deliveryTime").setFormatter(dateFormatter);
}
Collection Formatter
Collection formatter is used to format a collection into a string where commas separate the elements of the collection.
Layout descriptor usage:
<table id="customersTable"
width="100%"
dataContainer="customersDc">
<columns>
<column id="favouriteBrands">
<formatter>
<collection/>
</formatter>
</column>
</columns>
</table>
Java code usage:
@Autowired
protected ApplicationContext applicationContext;
@Autowired
protected Table<Customer> customersTable;
@Subscribe
protected void onInit(InitEvent event) {
CollectionFormatter collectionFormatter = applicationContext.getBean(CollectionFormatter.class);
customersTable.getColumn("favouriteBrands").setFormatter(collectionFormatter);
}
Custom Formatter
You can use a custom Java class as a formatter. It should implement the Formatter
interface.
An example of declaring a custom formatter:
@Component("ui_CurrencyFormatter")
@Scope(BeanDefinition.SCOPE_PROTOTYPE)
public class CurrencyFormatter implements Formatter<BigDecimal> {
@Override
public String apply(BigDecimal value) {
return NumberFormat.getCurrencyInstance(Locale.getDefault()).format(value);
}
}
In a screen XML-descriptor, a custom formatter can be defined in a nested custom
element:
<column id="amount">
<formatter>
<custom bean="ui_CurrencyFormatter"/>
</formatter>
</column>
Example of creating a custom validator programmatically in a screen controller:
@Autowired
protected Table<Customer> customersTable;
@Subscribe
protected void onInit(InitEvent event) {
customersTable.getColumn("age").setFormatter(value -> value + " y.o.");
}