Getting Started with Search
This guide provides instructions on initiating Search functionality within your application.
Suppose you wish to introduce a few entities to your application and enable searching across their attributes.
To begin, follow these steps:
-
Include Search in your project following the guidelines in the installation section.
-
Configure connection based on the service’s location.
Create Data Model and Views
Let’s create the classes listed below:
-
The
Status
enumeration with theSILVER
andGOLD
values. -
The
Customer
entity with the fields:-
firstName
withString
type -
lastName
withString
type -
status
withStatus
type -
card
withFileRef
type
-
-
The
Order
entity with the fields:-
date
withDate
type -
number
withString
type -
amount
withInteger
type -
product
withString
type -
customer
with association toCustomer
, many-to-one cardinality
-
Set up the instance name for the Order
entity to be displayed as the search result.
Create detail and list views for the Customer
and Order
entities.
Create Index Definition Interface
Next, it is necessary to establish an Index Definition - an interface outlining which entities and attributes are to be indexed. Suppose we intend to search orders based on their number, product name, customer status, or customer last name. Within the Index Definition, we will define a method to specify the required attributes.
@JmixEntitySearchIndex(entity = Order.class)
public interface OrderIndexDefinition {
@AutoMappedField(includeProperties =
{"number", "product", "customer.status", "customer.lastName"})
void orderMapping();
}
-
The interface should be annotated with
@JmixEntitySearchIndex
with the required parameterentity
. -
The interface can be named arbitrarily.
-
The
@AutoMappedField
annotation automatically maps requested properties. Here, we will utilize theincludeProperties
parameter to indicate the attributes to be indexed. -
The method can be named at your discretion.
Configure Index Naming
By default, index name is formed as <prefix><entity_name>
. Default prefix is search_index_
.
As you may utilize the same Elasticsearch/OpenSearch service across multiple projects, it is advisable to customize the default prefix for unique index names. To implement this change, add the following property to your application.properties
file:
jmix.search.search-index-name-prefix = demo_prefix_
As alternative way you can specify full index name in indexName
attribute of @JmixEntitySearchIndex
.
Configure Indexing Queue Processing
The Jmix application monitors data modifications but does not automatically synchronize them with search engine service. To ensure regular updates of indexes, simply add the Quartz add-on to the project. The Search add-on will leverage Quartz for scheduled processing of the indexing queue using default configurations.
Create Search View
-
Create an empty view named Search by utilizing the
Blank view
Studio template. -
Click Add Component in the actions panel, find the
SearchField
item, and then double-click it. -
The newly added
searchField
element will appear in both the Jmix UI structure panel and in the XML. You can configure attributes likeid
,height
,width
, etc., in the same way as it is done for other UI components.<search:searchField id="searchField"/>
Now, the view includes a text field for inputting the search term and a button to execute the search.
Test Searching in Application
Now, we are ready to launch and test the application.
Initially, add some instances of the Customer
and Order
entities.
Navigate to the Search view to locate customers with the Silver
status.
The search outcomes will appear in the Search results view.
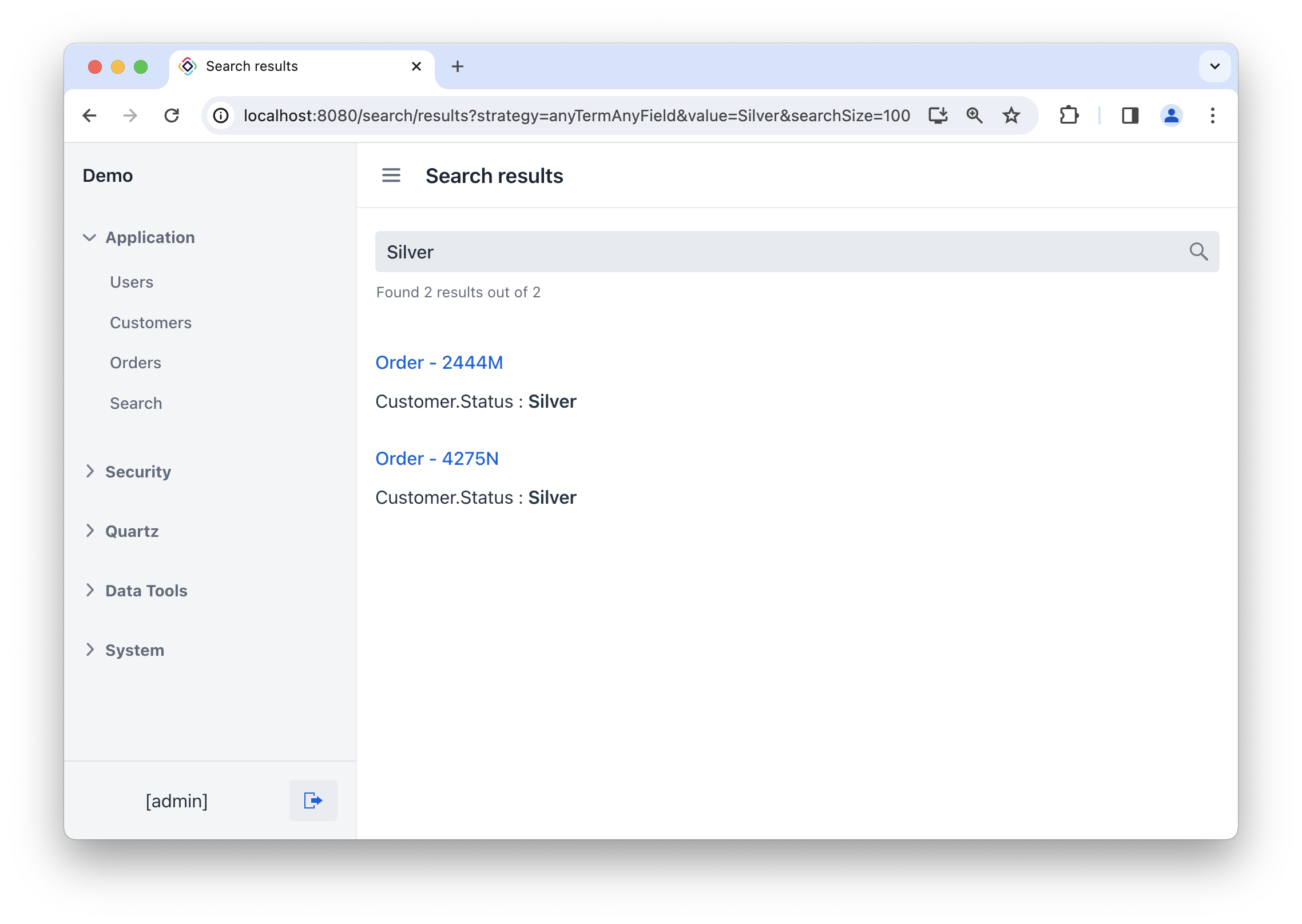
Clicking on a result will redirect you to the entity detail view.