tabSheet
tabSheet
is a container which allows switching between tabs. Several tabs help group content on the page.
-
XML element:
tabSheet
-
Java class:
JmixTabSheet
Basics
The contents of a tab is described by the tab
component. Each tab
can contain only one child component. Multiple components must be wrapped with a layout component, such as vbox.
<tabSheet id="tabSheet">
<tab id="tab1" label="Tab One">
<vbox>
<label text="Label One"/>
<textField placeholder="Text Field One"/>
</vbox>
</tab>
<tab id="tab2" label="Tab Two">
<label text="Label Two"/>
</tab>
<tab id="tab3" label="Tab Three">
<label text="Label Three"/>
</tab>
</tabSheet>
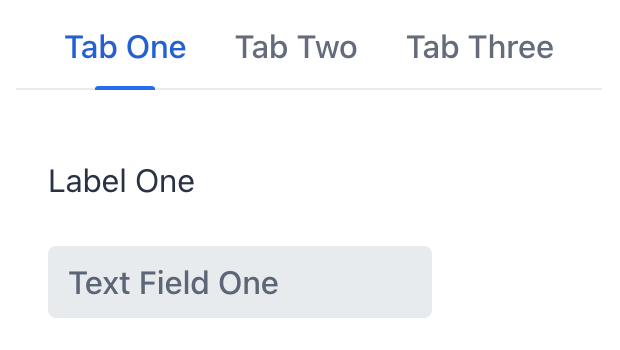
To add |
tabSheet
can have any practical number of tabs. If there are more tabs than the tab bar can fit, navigation buttons will appear.
Handlers
Чтобы сгенерировать заглушку обработчика в Jmix Studio, используйте вкладку Handlers панели инспектора Jmix UI, или команду Generate Handler, доступную на верхней панели контроллера экрана и через меню Code → Generate (Alt+Insert / Cmd+N). |
See Also
See Vaadin Docs for more information.