fileStorageUploadField
fileStorageUploadField
allows users to upload a file to the Jmix file storage.
-
XML element:
fileStorageUploadField
-
Java class:
FileStorageUploadField
Basics
The component can contain a label, a link to the uploaded file, and an upload button. When the upload button is clicked, a standard file picker dialog pops up, allowing the user to select a file.
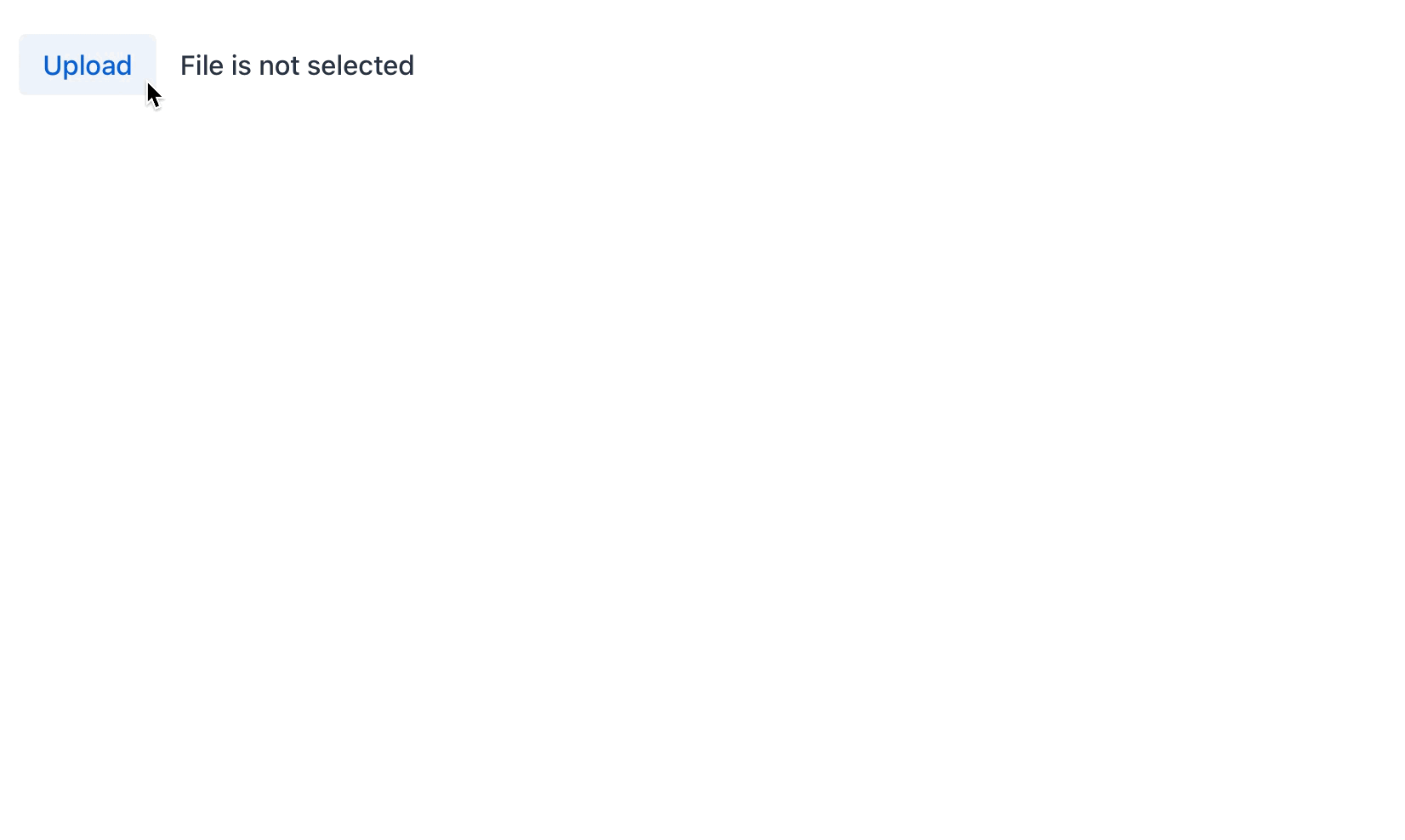
fileStorageUploadField
can work both without being bound to an entity attribute and with data binding.
Below we will show an example of using the component without data binding. You can use it to handle the uploaded file directly without associating it with any Jmix entity.
<fileStorageUploadField id="fileRefField"
acceptedFileTypes=".xlsx, .xls"
fileStoragePutMode="MANUAL"
fileNameVisible="true"/>
Then you can handle the uploaded file directly within the FileUploadSucceededEvent.
To store a file in an entity attribute as a byte array instead of To upload multiple files simultaneously, you should use the upload component instead of |
The uploaded file will be immediately stored in the default FileStorage . To control the saving of the file programmatically, use the fileStoragePutMode attribute.
|
Data Binding
fileStorageUploadField
allows users to upload a file to the file storage and link it to an entity attribute as a FileRef
object.
In the example below, the picture
attribute of the User
entity has the FileRef
type.
@Column(name = "PICTURE", length = 1024)
private FileRef picture;
<data>
<instance class="com.company.onboarding.entity.User" id="userDc">
<fetchPlan extends="_base"/>
<loader id="userDl"/>
</instance>
</data>
<layout>
<fileStorageUploadField dataContainer="userDc"
property="picture"
clearButtonVisible="true"
fileNameVisible="true"/>
</layout>
fileStorageUploadField
has a link to the container specified in the dataContainer
attribute; the property
attribute contains the name of the entity attribute that is displayed in fileStorageUploadField
.
Internationalization
All labels and messages in fileStorageUploadField
are configurable.
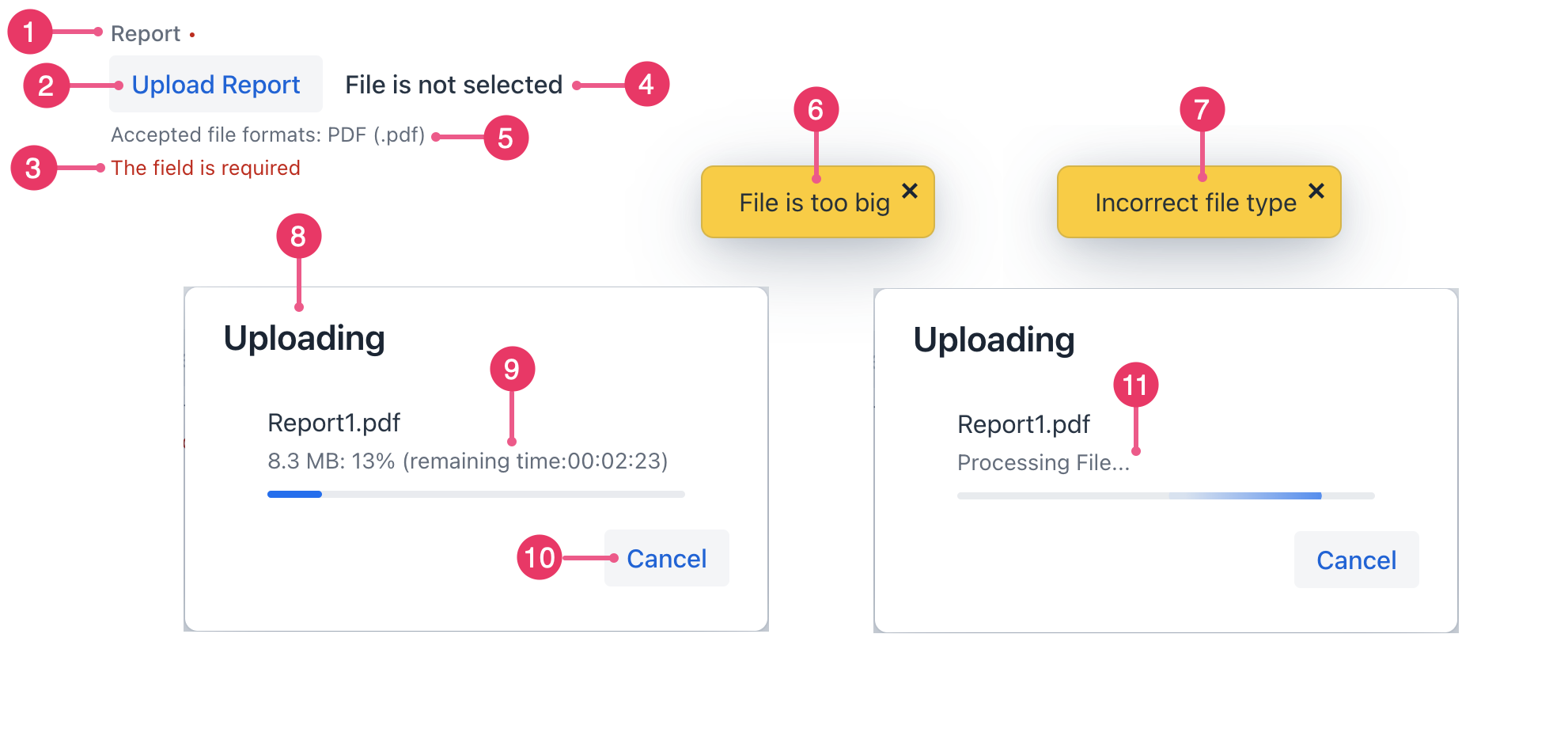
-
The label attribute.
-
The uploadText attribute.
-
The requiredMessage attribute.
-
The fileNotSelectedText attribute.
-
The helperText attribute.
-
The fileTooBigText attribute.
-
The incorrectFileTypeText attribute.
-
The uploadDialogTitle attribute.
-
The remainingTimeText attribute.
-
The uploadDialogCancelText attribute.
-
The processingStatusText attribute.
connectingStatusText
Defines the text of status in the upload dialog when connection is set.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
fileNotSelectedText
Sets the text that is shown when fileNameVisible="true" and the file is not uploaded. If not set, the default "File is not selected" text is shown.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
fileTooBigText
Sets the text of exception when the file size exceeds the maxFileSize value.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
incorrectFileTypeText
Sets the text of the message that is shown when the file extension is not included to the acceptedFileTypes attribute.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
processingStatusText
Sets the text of status in the upload dialog when a file is uploaded and processed.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
remainingTimeText
Sets the text in the upload dialog when it shows the remaining time.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
remainingTimeUnknownText
Sets the text in the upload dialog when it cannot calculate the remaining time.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
uploadDialogCancelText
Sets the text to the cancel button in the upload dialog.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
uploadDialogTitle
Sets the title of the upload dialog that is shown while uploading a file.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
uploadText
Sets the text that should be shown in the upload button.
The attribute value can either be the text itself or a key in the message bundle. In case of a key, the value should begin with the msg://
prefix.
Attributes
id - acceptedFileTypes - alignSelf - classNames - clearButtonAriaLabel - clearButtonVisible - colspan - connectingStatusText - css - dataContainer - dropAllowed - enabled - errorMessage - fileNameVisible - fileNotSelectedText - fileStorageName - fileStoragePutMode - fileTooBigText - height - helperText - incorrectFileTypeText - label - maxFileSize - maxHeight - maxWidth - minHeight - minWidth - processingStatusText - property - readOnly - remainingTimeText - remainingTimeUnknownText - required - requiredMessage - uploadDialogCancelText - uploadDialogTitle - uploadIcon - uploadText - visible - width
acceptedFileTypes
Specifies the types of files that the server accepts. The acceptable file formats are set using MIME type patterns or file extensions.
MIME types are widely supported, while file extensions are only implemented in certain browsers, so it should be avoided. |
<fileStorageUploadField dataContainer="userDc"
property="picture"
acceptedFileTypes="image/png, .png"/>
The acceptedFileTypes attribute filters the files displayed in the system file chooser dialog, ensuring users only select files with the allowed extensions.
|
clearButtonVisible
Sets whether the clear button should be shown after the file name. Works only if fileNameVisible="true".
The default value is false
.
dropAllowed
Sets whether the component supports dropping files for uploading.
The default value is true
.
File can only be dropped over the component’s Upload button. |
fileNameVisible
The fileNameVisible
attribute controls whether the name of the uploaded file is displayed next to the upload button. It is false
by default.
fileStorageName
Sets the name of FileStorage where the upload file will be placed. If not set, the default FileStorage
will be used.
fileStoragePutMode
Sets mode which determines when a file will be put into FileStorage. By default, the fileStoragePutMode
attribute is set to the IMMEDIATE
value. You can control the saving of the file programmatically if you set the fileStoragePutMode
attribute to the MANUAL
value:
<fileStorageUploadField id="manuallyControlledField"
dataContainer="userDc"
property="picture"
clearButtonVisible="true"
fileNameVisible="true"
fileStoragePutMode="MANUAL"/>
In the view controller, define the component itself, and the TemporaryStorage
interface. Then subscribe to the event of successful uploads:
@Autowired
private TemporaryStorage temporaryStorage;
@ViewComponent
private FileStorageUploadField manuallyControlledField;
@Autowired
private Notifications notifications;
@Subscribe("manuallyControlledField")
public void onManuallyControlledFieldFileUploadSucceeded(
final FileUploadSucceededEvent<FileStorageUploadField> event) {
Receiver receiver = event.getReceiver();
if (receiver instanceof FileTemporaryStorageBuffer) {
UUID fileId = ((FileTemporaryStorageBuffer) receiver)
.getFileData().getFileInfo().getId();
File file = temporaryStorage.getFile(fileId);
if (file != null) {
notifications.create("File is uploaded to temporary storage at "
+ file.getAbsolutePath())
.show();
}
FileRef fileRef = temporaryStorage.putFileIntoStorage(fileId, event.getFileName());
manuallyControlledField.setValue(fileRef);
notifications.create("Uploaded file: "
+ ((FileTemporaryStorageBuffer) receiver).getFileData().getFileName())
.show();
}
}
The component will upload the file to the temporary storage and invoke FileUploadSucceedEvent
.
The TemporaryStorage.putFileIntoStorage()
method moves the uploaded file from the temporary client storage to the default File Storage.
The ComponentValueChangeEvent is not fired automatically after uploading the fileStorageUploadField field with MANUAL put mode, because the FileRef value can be obtained only after uploading a file to the permanent file storage.
|
maxFileSize
Specifies the maximum file size in bytes allowed to upload. Notice that it is a client-side constraint, which will be checked before sending the request.
If MultipartProperties is available, the default value will be set from MultipartProperties#getMaxFileSize() that is equal to 1Mb. To increase maximum file size for all fields in the application use MultipartProperties#getMaxFileSize() property.
|
Handlers
AttachEvent - ComponentValueChangeEvent - DetachEvent - FileUploadFailedEvent - FileUploadFileRejectedEvent - FileUploadFinishedEvent - FileUploadProgressEvent - FileUploadStartedEvent - FileUploadSucceededEvent - statusChangeHandler - validator
Чтобы сгенерировать заглушку обработчика в Jmix Studio, используйте вкладку Handlers панели инспектора Jmix UI, или команду Generate Handler, доступную на верхней панели контроллера экрана и через меню Code → Generate (Alt+Insert / Cmd+N). |
FileUploadFailedEvent
io.jmix.flowui.kit.component.upload.event.FileUploadFailedEvent
is fired when FailedEvent
of Upload
is occurred. See Upload.addFailedListener(ComponentEventListener) for details.
@Subscribe("manuallyControlledField")
public void onManuallyControlledFieldFileUploadFailed(
final FileUploadFailedEvent<FileStorageUploadField> event) {
notifications.create("File upload error")
.show();
}
FileUploadFileRejectedEvent
io.jmix.flowui.kit.component.upload.event.FileUploadFileRejectedEvent
is fired when FileRejectedEvent
of Upload
is occurred. See Upload.addFileRejectedListener(ComponentEventListener) for details.
FileUploadFinishedEvent
io.jmix.flowui.kit.component.upload.event.FileUploadFinishedEvent
is fired when FinishedEvent
of Upload
is occurred. See Upload.addFinishedListener(ComponentEventListener) for details.
FileUploadProgressEvent
io.jmix.flowui.kit.component.upload.event.FileUploadProgressEvent
is fired when ProgressUpdateEvent
of Upload
is occurred. See Upload.addProgressListener(ComponentEventListener) for details.
FileUploadStartedEvent
io.jmix.flowui.kit.component.upload.event.FileUploadStartedEvent
is fired when StartedEvent
of Upload
is occurred. See Upload.addStartedListener(ComponentEventListener) for details.
FileUploadSucceededEvent
io.jmix.flowui.kit.component.upload.event.FileUploadSucceededEvent
is fired when the Upload
component’s SucceededEvent
occurs, meaning the upload has been successfully received. See Upload.addSucceededListener(ComponentEventListener) for details.
Here is an example of using fileStorageUploadField
for .xls
и .xlsx
files, and then processing those files in FileUploadSucceededEvent
.
@Subscribe("fileRefField")
public void onFileRefFieldFileUploadSucceeded(
final FileUploadSucceededEvent<FileStorageUploadField> event) {
if (event.getReceiver() instanceof FileTemporaryStorageBuffer buffer) {
UUID fileId = buffer.getFileData().getFileInfo().getId();
log.info("FileId: " + fileId);
File file = temporaryStorage.getFile(fileId); (1)
log.info("File from temp storage: " + file);
try { (2)
List<String> lines = FileUtils.readLines(file, StandardCharsets.UTF_8);
for (String line : lines) {
log.info("Read line: " + line);
}
} catch (IOException e) {
throw new RuntimeException(e);
}
temporaryStorage.deleteFile(fileId); (3)
log.info("File is deleted from temp storage: " + file);
}
}
1 | Get local file from the temporary storage using identifier provided by the upload event. |
2 | Process the file data. |
3 | Remove the uploaded file. In a real-world application you would move the file to FileStorage here using the temporaryStorage.putFileIntoStorage() method. |
You can also find an example of FileUploadSucceededEvent
in the fileStoragePutMode description.