Style
Style for PointFeature
To change the style for a PointFeature, you typically define a PointStyle
object with the desired appearance properties like image
, fill
, stroke
, etc. and then apply that style to the PointFeature
.
private PointFeature createStyledPoint() {
return new PointFeature(GeometryUtils.createPoint(22, 25))
.withStyles(
new PointStyle()
.withImage(new CircleStyle()
.withRadius(7)
.withFill(new Fill("#E7003E"))
.withStroke(new Stroke()
.withWidth(2.0)
.withColor("#710067")))
.build());
}
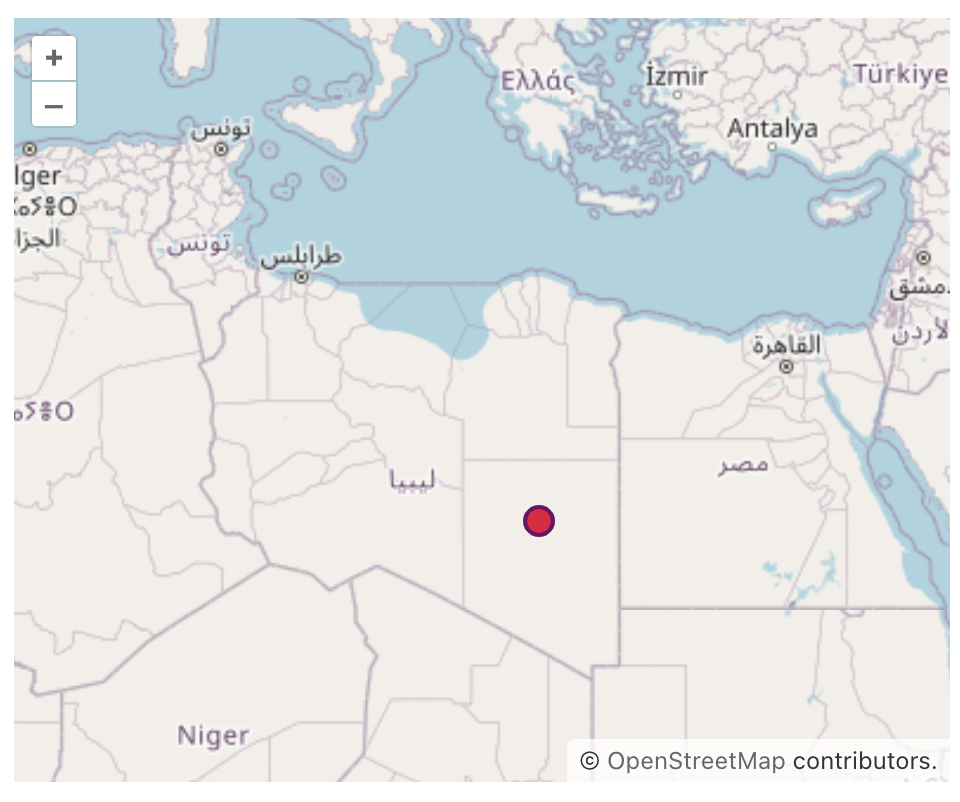
Using text instead of an image for the PointFeature
allows you to display textual labels directly on the map at specified coordinates, providing clarity and context to the displayed data.
When utilizing text for PointFeature
, you can customize the appearance of the text by adjusting properties like font
, fill
, textAlign
, etc.
The example below demonstrates using textual label instead of an image for the PointFeature
:
private PointFeature createTextPoint() {
return new PointFeature(GeometryUtils.createPoint(15, 15))
.withStyles(
new PointStyle()
.withText(new TextStyle()
.withText("Africa")
.withFont("20px sans-serif")
.withFill(new Fill("#5E4BD8"))
.withStroke(new Stroke()
.withWidth(2.)
.withColor("#A368D5")))
.build());
}
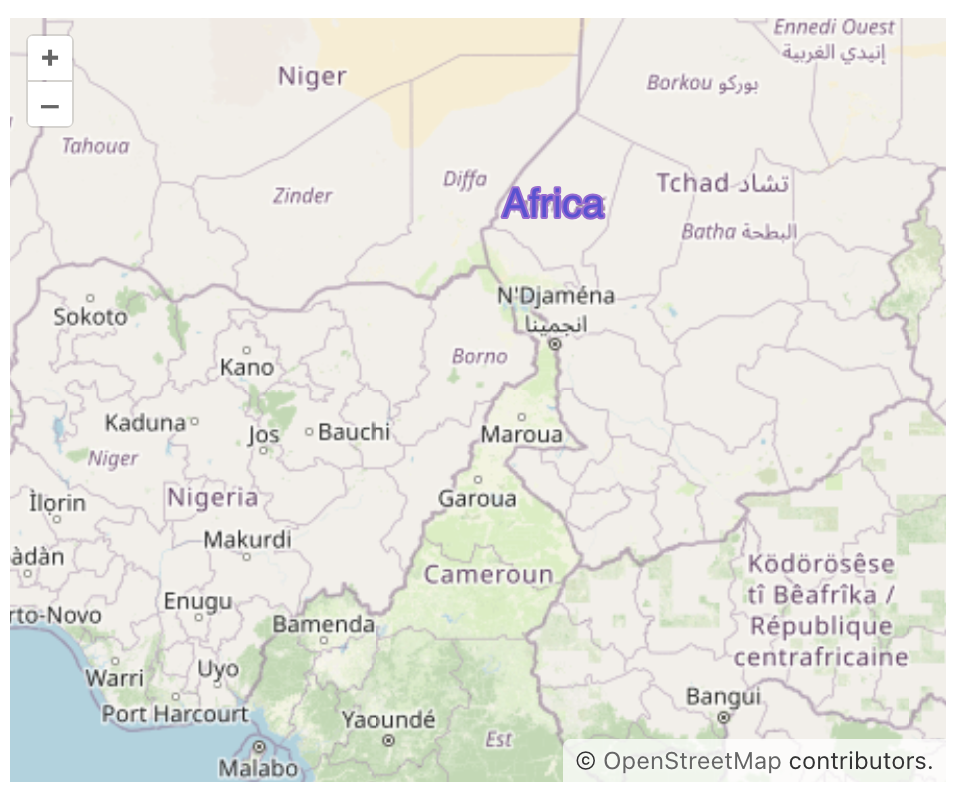
Style for MarkerFeature
To change the marker icon, refer the following example:
private MarkerFeature createStyledMarker() {
MarkerFeature feature = new MarkerFeature(GeometryUtils.createPoint(20, 20));
feature.removeAllStyles();
return feature.withStyles(new Style()
.withImage(new IconStyle()
.withSrc("icons/icon.png")
.withScale(0.05)));
}
Additionally, an example of customizing marker icons based on the geo-object’s attributes is provided in the Use Custom Markers section.
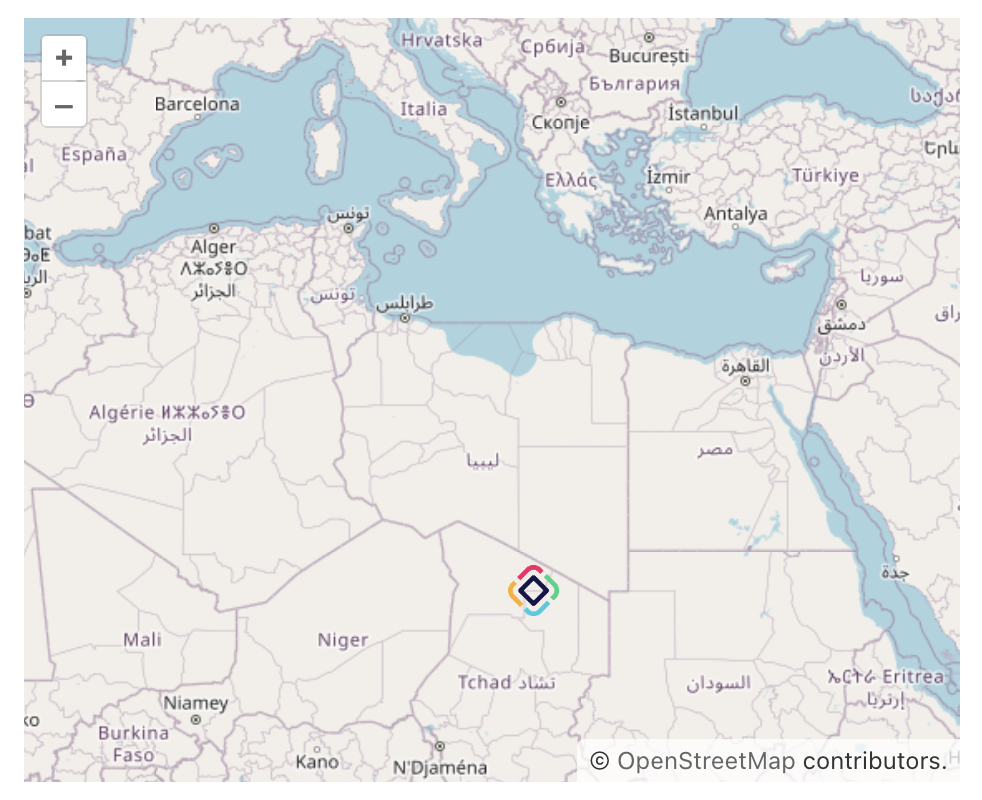
Style for LineStringFeature
To change the style for a LineStringFeature
, you typically define a LineStringStyle
object with the desired appearance properties like stroke
, fill
, etc., and then apply that style to the LineStringFeature
.
private LineStringFeature createStyledLineString() {
LineString lineString = geometries.createLineString(new Coordinate[]{
new Coordinate(13, 20),
new Coordinate(13, 32),
new Coordinate(25, 17)});
return new LineStringFeature(lineString)
.withStyles(
new LineStringStyle()
.withStroke(new Stroke()
.withWidth(3.)
.withColor("#F60018"))
.build());
}
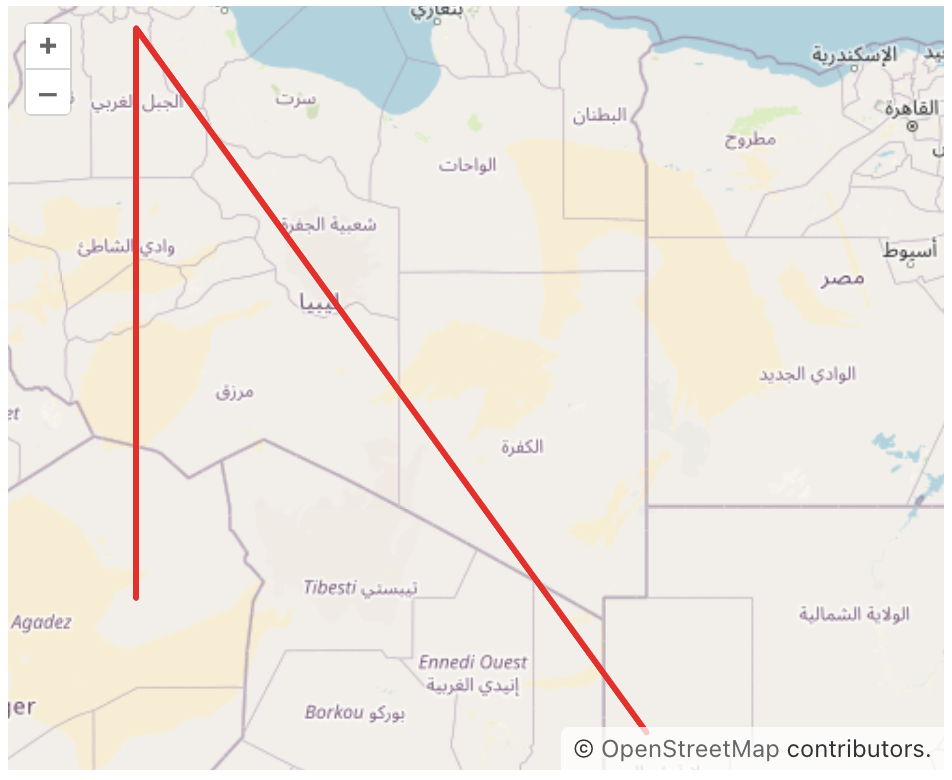
Style for PolygonFeature
Changing the style for a PolygonFeature
involves defining a new PolygonStyle
with desired properties like fill
, stroke
, etc., and applying this style to the PolygonFeature
.
private PolygonFeature createStyledPolygon() {
LinearRing shell = geometries.createLinearRing(new Coordinate[]{
new Coordinate(1.2457020544488762, 42.476628901048684),
new Coordinate(-0.054875980233204155, 52.77260344863316),
new Coordinate(29.858418817454655, 46.105591288830624),
new Coordinate(1.2457020544488762, 42.476628901048684),
});
return new PolygonFeature(geometries.createPolygon(shell))
.withStyles(
new PolygonStyle()
.withFill(new Fill("rgba(1, 147, 154, 0.2)"))
.withStroke(new Stroke()
.withWidth(3.)
.withColor("#123EAB"))
.build());
}
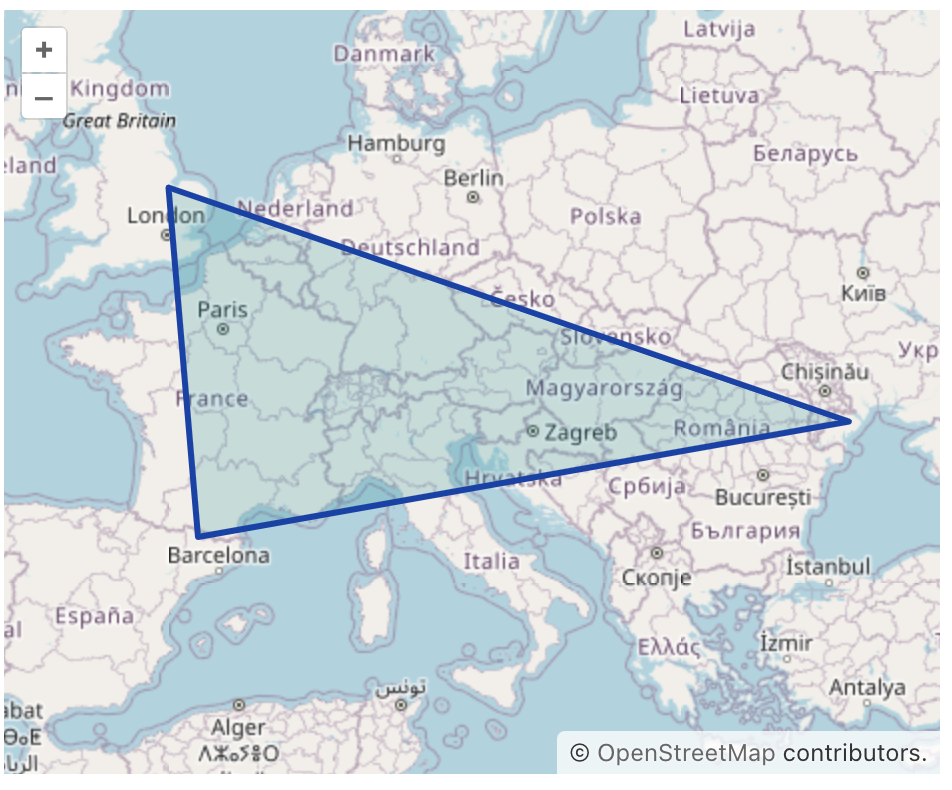