Creating Notifications
You can create notifications via administration UI or programmatically in code.
Administration UI
After the add-on is added to your application, the main menu contains the Notifications item. The Notifications browser provides a list of created notifications and buttons for managing them.
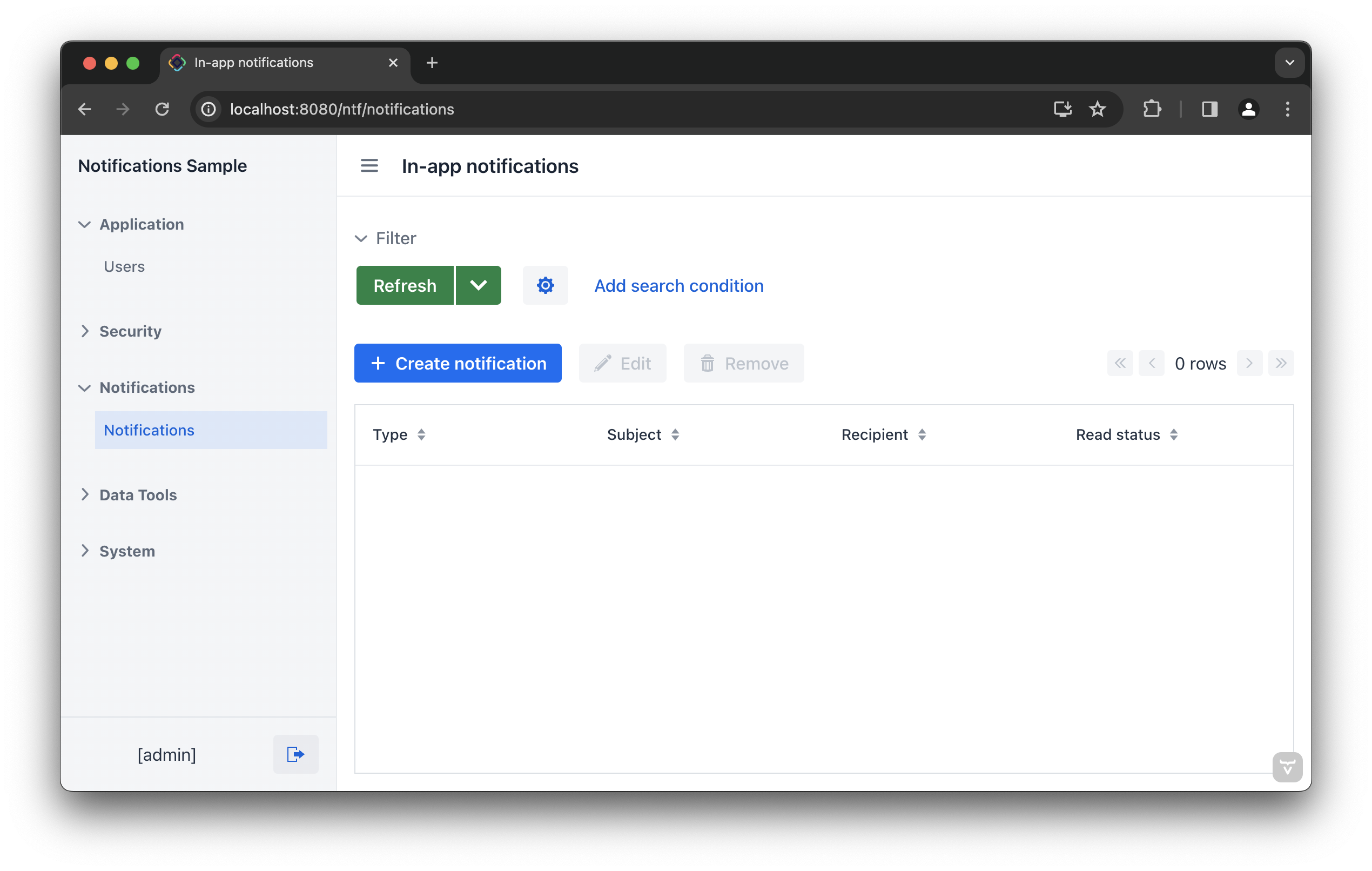
The notification editor appears after clicking the Create new notification button.
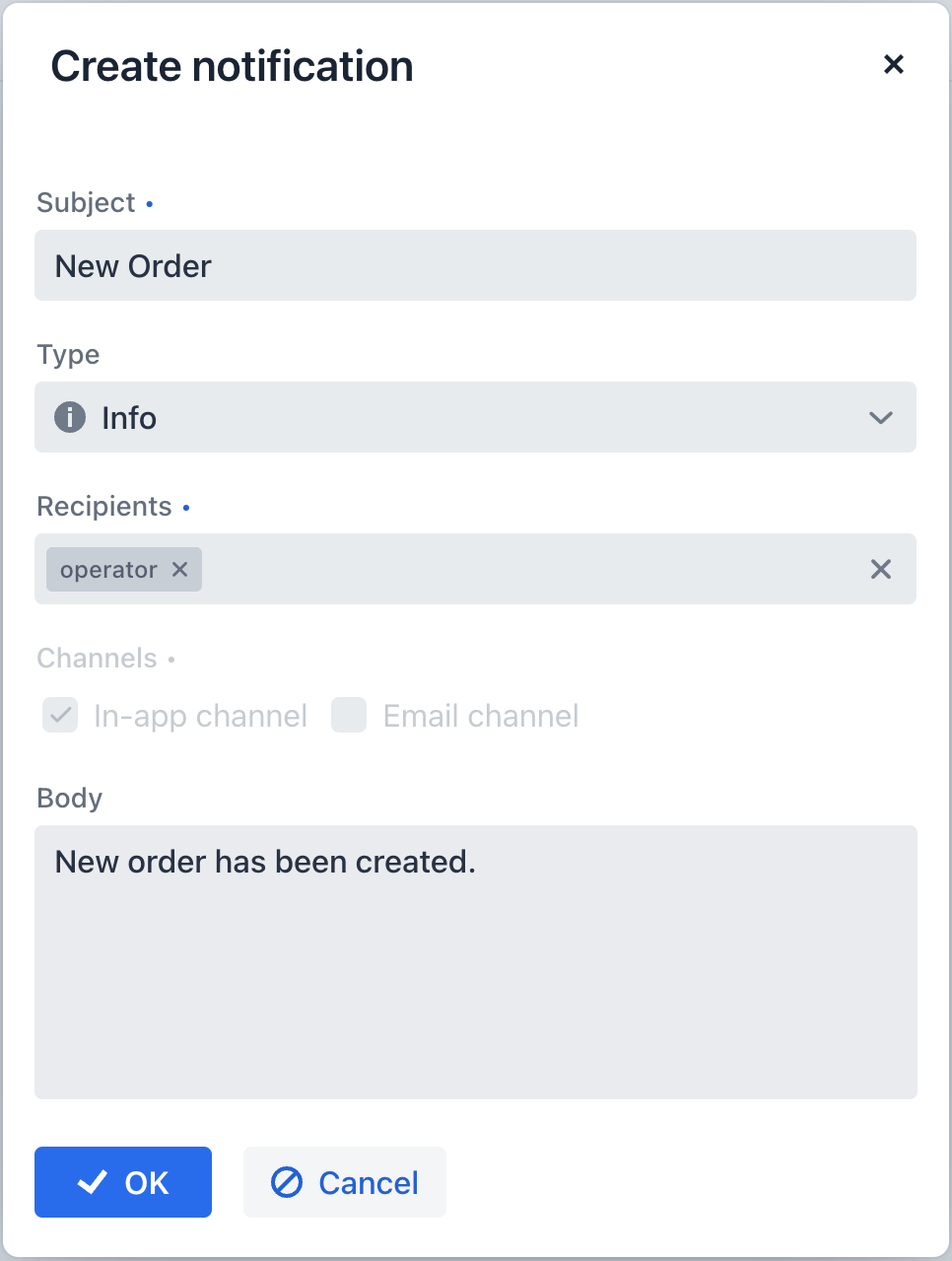
The dialog provides the following fields to fill in:
-
Subject – a subject of the notification.
-
Type – a type of notification. There are no default notification types. See the configuration section to create types.
-
Recipients – list of recipients of the notification. Start typing a username in the field, and select the appropriate users from the list.
-
Channels – a channel used for notification.
-
In-app channel – a user will receive the notification in the application UI.
-
Email channel – a user will receive an email with the notification. (available with Email add-on.)
-
-
Body – body of the notification.
Programmatic Creation
The NotificationManager service provides methods to send notifications to users.
In the example below, a notification is sent to the user every time a new order is created.
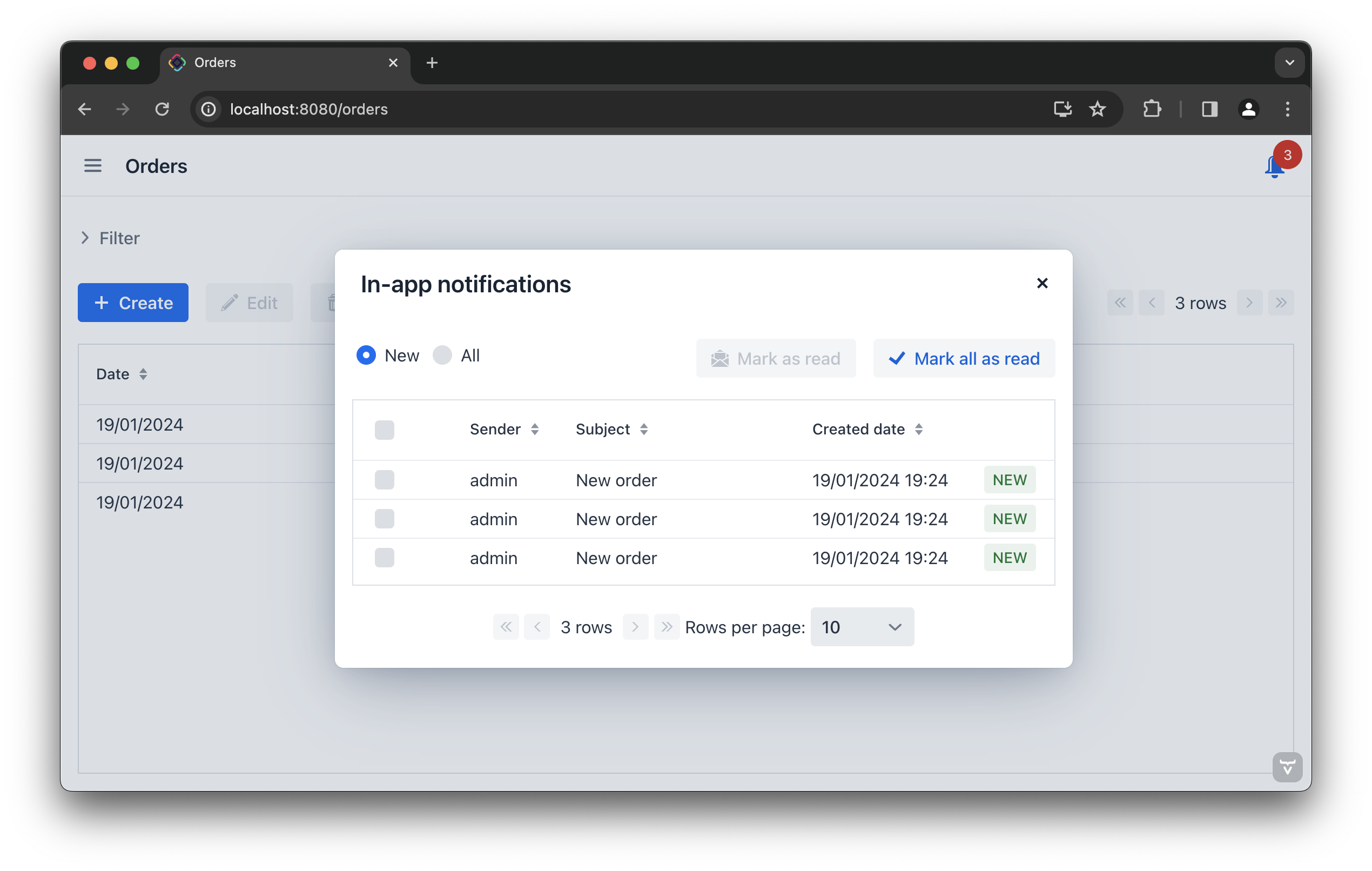
private boolean justCreated;
@ViewComponent
private TypedTextField<Integer> numberField;
@Autowired
protected NotificationManager notificationManager;
@Subscribe
public void onInitEntity(InitEntityEvent<Order> event) {
justCreated = true;
}
@Subscribe(target = Target.DATA_CONTEXT)
public void onPostSave(final DataContext.PostSaveEvent event) { (1)
if (justCreated) { (2)
notificationManager.createNotification() (3)
.withSubject("New order")(4)
.withRecipientUsernames("admin") (5)
.toChannelsByNames("in-app") (6)
.withContentType(ContentType.PLAIN) (7)
.withBody("A new order with number " + numberField.getValue()+ " is created.") (8)
.send(); (9)
}
}
1 | This method is invoked after saving changes. |
2 | Checks if this entity is newly created. |
3 | Initiates NotificationRequestBuilder that creates a new NotificationRequest object. |
4 | Defines the subject of the notification. |
5 | Sets the recipient by the provided username. |
6 | Sets notification channels by provided names. The in-app and email are available. |
7 | Set notification body content type. |
8 | Sets notification body. |
9 | Creates and sends the notification request. |
Click Code Snippets in the actions panel to generate code for sending notifications. |