loginForm
A form for entering authentication credentials.
-
XML element:
loginForm
-
Java class:
JmixLoginForm
Basics
Basic loginForm
includes username and password fields and a button that the user clicks to attempt logging in. The form can also include optional elements such as a locales combobox, "Remember me" checkbox and "Forgot password" button.
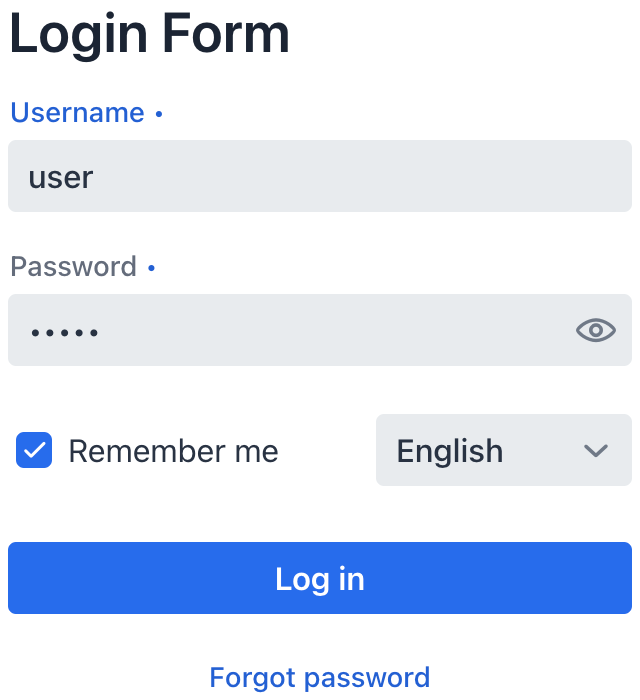
Labels and Messages
Include the form
element to specify the form’s title, input field labels, and button text.
Values in the form can be strings of text or keys in the message bundle. |
<loginForm>
<form title="Welcome"
username="Username or email"
password="Password"
rememberMe="Remember me"
submit="Log in"
forgotPassword="Forgot password"/>
<errorMessage title="Login failed"
message="Check that you have entered the correct username and password and try again"/>
<additionalInformation message="Additional information"/>
</loginForm>
The example above also adds an optional errorMessage
element to display a message when login fails and an additionalInformation
element to provide relevant details about the login process.
Authentication
The standard login view will include the necessary logic for authenticating the user with the application:
@ViewComponent
private JmixLoginForm login; (1)
@Subscribe("login")
public void onLogin(final LoginEvent event) {
try {
loginViewSupport.authenticate(
AuthDetails.of(event.getUsername(), event.getPassword())
.withLocale(login.getSelectedLocale()) (2)
.withRememberMe(login.isRememberMe()) (3)
);
} catch (final BadCredentialsException | DisabledException | LockedException | AccessDeniedException e) {
log.info("Login failed", e);
event.getSource().setError(true);
}
}
1 | Inject the loginForm to access it by id in the controller class. |
2 | Applies the selected locale for the application. |
3 | Checks whether to remember the user between sessions based on the checkbox value. |
Exceptions Handling
To help users identify login issues, extend the default logic to include informative messages for each exception type:
@Subscribe("login")
public void onLogin(final AbstractLogin.LoginEvent event) {
try {
loginViewSupport.authenticate(
AuthDetails.of(event.getUsername(), event.getPassword())
.withLocale(login.getSelectedLocale())
.withRememberMe(login.isRememberMe())
);
}
catch (final BadCredentialsException | DisabledException | LockedException | AccessDeniedException e) {
if (e instanceof LockedException) {
JmixLoginI18n loginI18n = createLoginI18n();
loginI18n.getErrorMessage().setMessage(e.getMessage()); (1)
login.setI18n(loginI18n);
} else {
login.setI18n(createLoginI18n());
}
log.warn("Login failed for user '{}': {}", event.getUsername(), e.toString()); (2)
event.getSource().setError(true);
}
}
1 | Displays the error message for a login failure based on the exception, specifically a LockedException , which indicates that the account is temporarily locked. |
2 | Logs a warning message indicating that a login attempt failed for a specific user. |
Locale
The login form can include a combobox that allows the user to select their preferred locale. To make the preferred option available, you need to set up the locale in your application. Once an additional locale is set up, it will appear in the list.
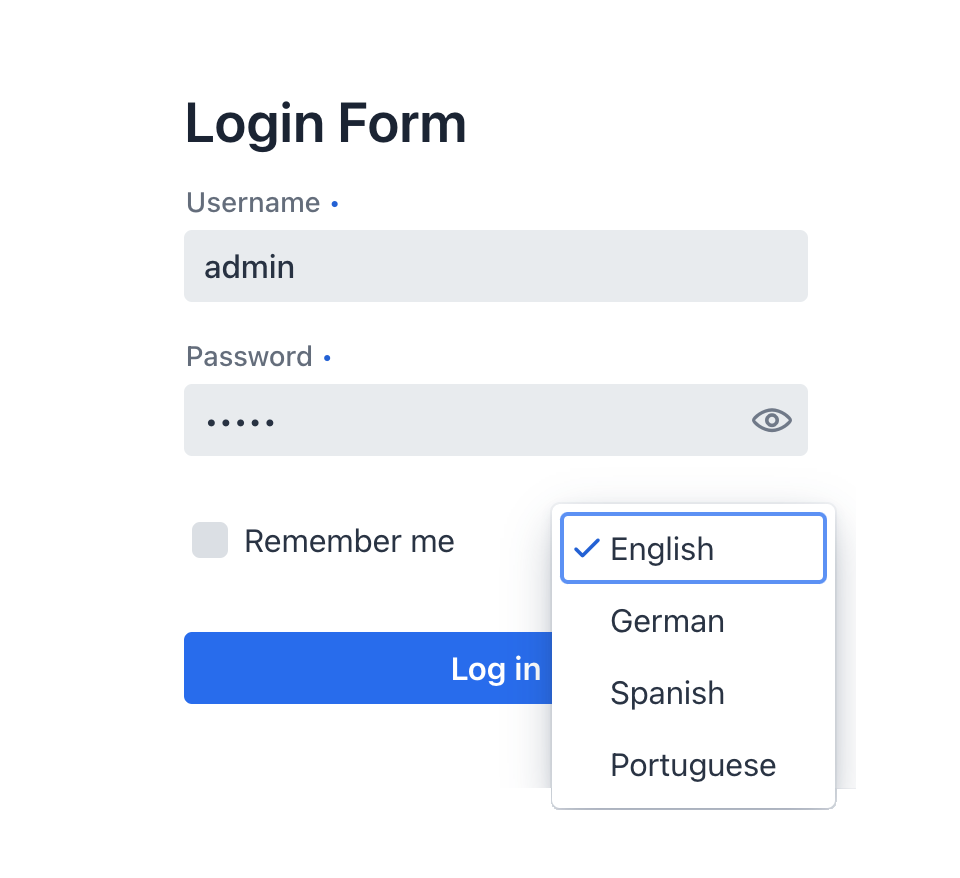
Whenever the user selects a new locale from the list, the LocaleChangedEvent is fired. By creating a handler for this event, you can ensure that the login form messages will update immediately without requiring a page reload. This is how it is done on the standard login view:
@Override
public void localeChange(final LocaleChangeEvent event) {
UI.getCurrent().getPage().setTitle(messageBundle.getMessage("LoginView.title"));
final JmixLoginI18n loginI18n = JmixLoginI18n.createDefault();
final JmixLoginI18n.JmixForm form = new JmixLoginI18n.JmixForm();
form.setTitle(messageBundle.getMessage("loginForm.headerTitle"));
form.setUsername(messageBundle.getMessage("loginForm.username"));
form.setPassword(messageBundle.getMessage("loginForm.password"));
form.setSubmit(messageBundle.getMessage("loginForm.submit"));
form.setForgotPassword(messageBundle.getMessage("loginForm.forgotPassword"));
form.setRememberMe(messageBundle.getMessage("loginForm.rememberMe"));
loginI18n.setForm(form);
final LoginI18n.ErrorMessage errorMessage = new LoginI18n.ErrorMessage();
errorMessage.setTitle(messageBundle.getMessage("loginForm.errorTitle"));
errorMessage.setMessage(messageBundle.getMessage("loginForm.badCredentials"));
loginI18n.setErrorMessage(errorMessage);
login.setI18n(loginI18n);
}
Styling the Login View
You can use CSS styles to enhance the appearance and layout of the login view in your application. One way to improve the view is by adding a background image. This image will visually confirm to the user that they are logging into the right app.
Attributes
id - alignSelf - classNames - colspan - css - enabled - forgotPasswordButtonVisible - localesVisible - rememberMeVisible - visible
Handlers
AttachEvent - DetachEvent - ForgotPasswordEvent - LocaleChangedEvent - LoginEvent - RememberMeChangedEvent - localeItemLabelGenerator
Чтобы сгенерировать заглушку обработчика в Jmix Studio, используйте вкладку Handlers панели инспектора Jmix UI, или команду Generate Handler, доступную на верхней панели контроллера экрана и через меню Code → Generate (Alt+Insert / Cmd+N). |
ForgotPasswordEvent
ForgotPasswordEvent
is sent when the Forgot password button is clicked. Add a handler to this event to provide users with password recovery instructions.
LocaleChangedEvent
LocaleChangedEvent
is sent when a different locale is selected. A handler for this event may be used to update labels and messages in the form to be of selected locale.
RememberMeChangedEvent
RememberMeChangedEvent
is sent when the state of the Remember me checkbox changes.
localeItemLabelGenerator
Customizes names for locales in the list. For example, instead of using the full language name for each locale, you can use a three-letter uppercase abbreviation.
@Install(to = "login", subject = "localeItemLabelGenerator")
private String loginLocaleItemLabelGenerator(final Locale locale) {
return locale.getISO3Language().toUpperCase();
}
See Also
See Vaadin Docs for more information.