textField
Поле ввода для редактирования текста или значений произвольных типов данных.
-
XML-элемент:
textField
-
Java-класс:
TypedTextField
Основы
Компонент textField
является типизированным, что позволяет работать с вводом различных типов данных. Вы можете задать его тип, привязав компонент к атрибуту сущности определённого типа или явно указав тип с помощью атрибута datatype.
<textField id="nameField"
label="Name"
datatype="string"
clearButtonVisible="true"
themeNames="always-float-label"
helperText="msg://textField.helper" placeholder="Test placeholder"/>
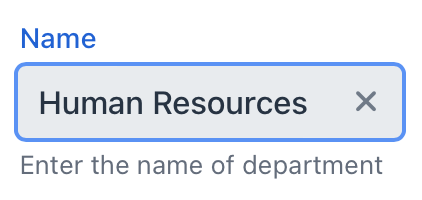
Дополнительные методы для работы с типизированными значениями включают setTypedValue()
и getTypedValue()
. Эти методы полезны, когда необходимо установить или получить значения конкретного типа, такие как Integer
или Long
.
При обработке изменений значений компонента рекомендуется использовать TypedValueChangeEvent вместо ComponentValueChangeEvent. Такой подход гарантирует корректность типа значения и исключает необходимость дополнительных преобразований или проверок.
Привязка данных
Для создания textField
, связанного с данными, используйте атрибуты dataContainer и property.
<data>
<instance class="com.company.onboarding.entity.Department"
id="departmentDc">
<fetchPlan extends="_base"/>
<loader id="departmentDl"/>
</instance>
</data>
<layout>
<textField dataContainer="departmentDc"
property="name"/>
</layout>
В приведённом выше примере экран описывает контейнер данных departmentDc
для сущности Department
, которая содержит атрибут name
. Компонент textField
связан с контейнером через атрибут dataContainer
; атрибут property
содержит имя атрибута сущности, отображаемого в textField
Варианты оформления
Используйте атрибут themeNames для настройки выравнивания текста, расположения вспомогательного текста и размера компонента.
Выравнивание
Доступны три варианта выравнивания: align-left
(по умолчанию), align-right
, align-center
.

XML код
<textField themeNames="align-left"/>
<textField themeNames="align-center"/>
<textField themeNames="align-right"/>
Положение вспомогательного текста
Установка значения helper-above-field
перемещает вспомогательный текст из стандартного положения под полем на позицию над ним.
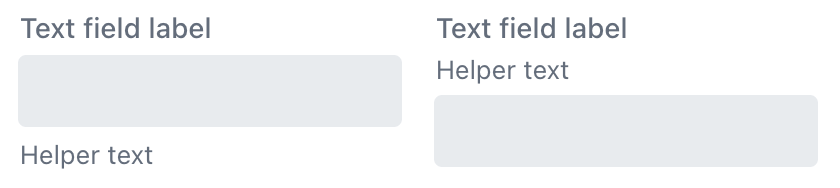
XML код
<textField label="Text field label" helperText="Helper text"/>
<textField themeNames="helper-above-field" label="Text field label" helperText="Helper text"/>
Атрибуты
id - alignSelf - allowedCharPattern - ariaLabel - ariaLabelledBy - autocapitalize - autocomplete - autocorrect - autofocus - autoselect - classNames - clearButtonVisible - colspan - css - dataContainer - datatype - enabled - errorMessage - focusShortcut - height - helperText - label - maxHeight - maxLength - maxWidth - minHeight - minLength - minWidth - pattern - placeholder - property - readOnly - required - requiredMessage - themeNames - trimEnabled - title - value - valueChangeMode - valueChangeTimeout - visible - width
autoselect
При значении true
текст в поле будет автоматически выделяться при получении фокуса. При false
- не будет.
trimEnabled
Если true
, компонент удаляет пробелы в начале и в конце введённой строки. Например, если пользователь вводит " aaa bbb ", значение поля, сохраняемое в атрибут связанной сущности, будет "aaa bbb".
Вы можете отключить удаление пробелов, установив trimEnabled
в false.
Значение по умолчанию для этого атрибута для всего приложения можно установить с помощью свойства приложения jmix.ui.component.default-trim-enabled.
value
Определяет значение textField
.
При невозможности преобразования значения к указанному типу данных будет показано стандартное сообщение об ошибке конвертации.
Обработчики
AttachEvent - BlurEvent - ClientValidatedEvent - ComponentValueChangeEvent - CompositionEndEvent - CompositionStartEvent - CompositionUpdateEvent - DetachEvent - FocusEvent - InputEvent - KeyDownEvent - KeyPressEvent - KeyUpEvent - statusChangeHandler - TypedValueChangeEvent - validator
Чтобы сгенерировать заглушку обработчика в Jmix Studio, используйте вкладку Handlers панели инспектора Jmix UI, или команду Generate Handler, доступную на верхней панели контроллера экрана и через меню Code → Generate (Alt+Insert / Cmd+N). |
validator
Добавляет экземпляр валидатора к компоненту. Валидатор должен выбрасывать ValidationException
при недопустимом значении.
@Install(to = "zipField", subject = "validator")
protected void zipFieldValidator(Integer value) {
if (value != null && String.valueOf(value).length() != 6)
throw new ValidationException("Zip must be of 6 digits length");
}
Смотрите также
Смотрите документацию Vaadin для получения дополнительной информации.